Unleashing the Power of React Server Components: A Game-Changing Tech Trend in JavaScript
In the ever-evolving landscape of JavaScript, a revolutionary trend is gaining momentum and reshaping the way developers approach building web applications. Enter React Server Components, a cutting-edge technology that promises to elevate the performance, flexibility, and scalability of JavaScript applications.
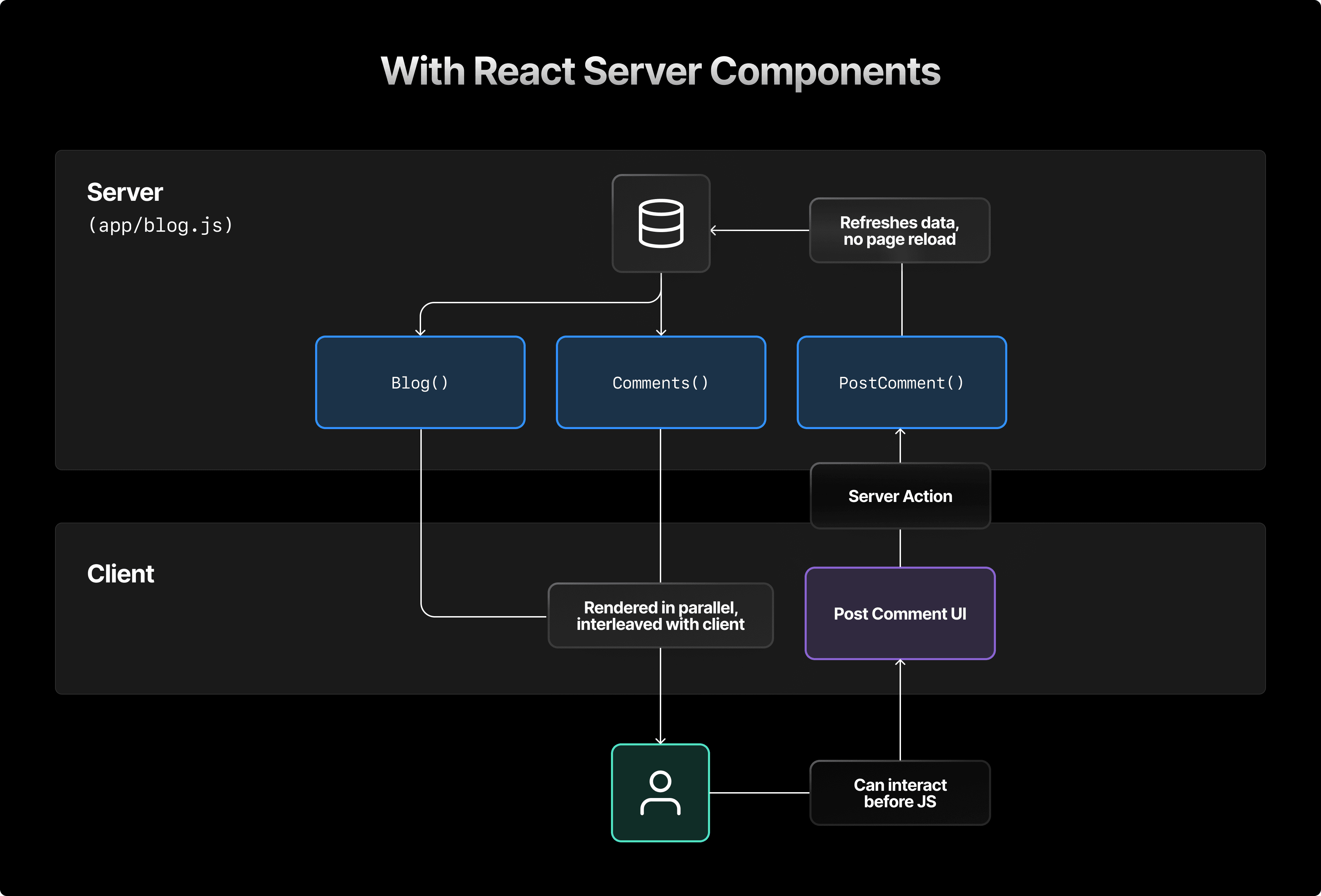
Server-Side Rendering Paradigm
Traditionally, front-end development has relied on client-side rendering, where the browser takes on the heavy lifting of rendering user interfaces. However, React Server Components take a different approach by introducing a server-side rendering paradigm. This means that instead of relying solely on the client, components can be rendered on the server and sent directly to the browser, resulting in faster initial loads and improved overall performance.
Dynamic Loading for Improved Efficiency
One of the key advantages of React Server Components is their ability to dynamically load components on the server, enabling a more efficient rendering process. This is particularly beneficial for large-scale applications with complex user interfaces, as it allows developers to selectively render components on the server based on specific user interactions. The result is a more responsive and seamless user experience, especially on devices with limited processing power.
Granular Code Splitting
Furthermore, React Server Components offer a significant improvement in code splitting. With traditional client-side rendering, code splitting often involves loading entire JavaScript bundles, which can impact performance. React Server Components, on the other hand, enable more granular code splitting by loading only the components needed for a specific page or interaction. This not only reduces the initial load time but also ensures that users only download the code necessary for their immediate needs.
// React Server Component
// File: MyServerComponent.js
import { serverRender } from 'react-dom/server';
function MyServerComponent() {
return (
<div>
<h1>Hello, React Server Component!</h1>
<p>This content is rendered on the server.</p>
</div>
);
}
// Server-side rendering function
export const render = serverRender(MyServerComponent);
In this example, we have a MyServerComponent that represents a simple React component. The crucial part is the render function, which uses the serverRender function from the react-dom/server module to render the component on the server.
Now, let's see how you can use this server-rendered component on the client side:
// Client-side React Component
// File: App.js
import React from 'react';
import { hydrate } from 'react-dom';
import MyServerComponent from './MyServerComponent';
function App() {
return (
<div>
<h1>Client-Side React Component</h1>
<MyServerComponent />
</div>
);
}
// Hydrate the server-rendered component on the client side
hydrate(<App />, document.getElementById('root'));
In this client-side example, we use the hydrate function from react-dom to attach event listeners and preserve the server-rendered markup. This combination of server-rendered and client-hydrated components helps improve the initial load time and enhances overall application performance.
Facilitating Front-End and Back-End Collaboration
Another noteworthy aspect of this trend is the potential for better collaboration between front-end and back-end developers. React Server Components allow both teams to work on the same components, promoting code reusability and streamlining the development process. This collaborative approach is particularly valuable in large projects where efficient communication and coordination between teams are essential.
Challenges and Considerations
As with any emerging technology, there are challenges and considerations to address when adopting React Server Components. Developers need to carefully plan and structure their applications to fully leverage the benefits of server-side rendering. Additionally, there may be a learning curve for those familiar with traditional client-side rendering approaches.
Conclusion
In conclusion, React Server Components represent a transformative shift in JavaScript development, offering enhanced performance, efficient code splitting, and improved collaboration between front-end and back-end teams. As developers continue to explore and integrate this trend into their workflows, we can anticipate a new era of web applications that are faster, more scalable, and built with a heightened focus on user experience.